Course overview
- Provider
- Udemy
- Course type
- Paid course
- Level
- All Levels
- Duration
- 11 hours
- Lessons
- 107 lessons
- Certificate
- Available on completion
- Course author
- Holczer Balazs
-
- Understanding recursion
- Understand backtracking
- Understand dynamic programming
- Understand divide and conquer methods
- Implement 15+ algorithmic problems from scratch
- Improve your problem solving skills and become a stronger developer
Description
This course is about the fundamental concepts of algorithmic problems focusing on recursion, backtracking, dynamic programming and divide and conquer approaches. As far as I am concerned, these techniques are very important nowadays, algorithms can be used (and have several applications) in several fields from software engineering to investment banking or R&D.
Section 1 - RECURSION
what are recursion and recursive methods
stack memory and heap memory overview
what is stack overflow?
Fibonacci numbers
factorial function
tower of Hanoi problem
Section 2 - SEARCH ALGORITHMS
linear search approach
binary search algorithm
Section 3 - SELECTION ALGORITHMS
what are selection algorithms?
Hoare's algorithm
how to find the k-th order statistics in O(N) linear running time?
quickselect algorithm
median of medians algorithm
the secretary problem
Section 4 - BACKTRACKING
what is backtracking?
n-queens problem
Hamiltonian cycle problem
coloring problem
knight's tour problem
maze problem
Section 5 - DYNAMIC PROGRAMMING
what is dynamic programming?
knapsack problem
rod cutting problem
subset sum problem
Section 6 - OPTIMAL PACKING
what is optimal packing?
bin packing problem
Section 7 - DIVIDE AND CONQUER APPROACHES
what is the divide and conquer approach?
dynamic programming and divide and conquer method
how to achieve sorting in O(NlogN) with merge sort?
the closest pair of points problem
Section 8 - COMMON INTERVIEW QUESTIONS
top interview questions (Google, Facebook and Amazon)
anagram problem
palindrome problem
integer reversion problem
dutch national flag problem
trapping rain water problem
In each section we will talk about the theoretical background for all of these algorithms then we are going to implement these problems together from scratch in Python.
Thanks for joining the course, let's get started!
Similar courses
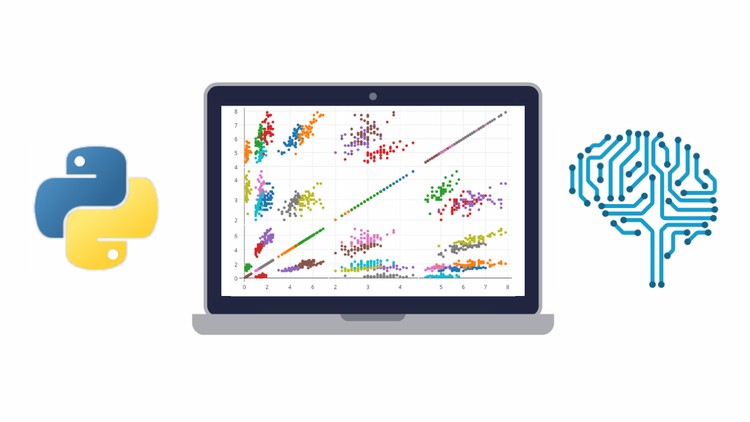

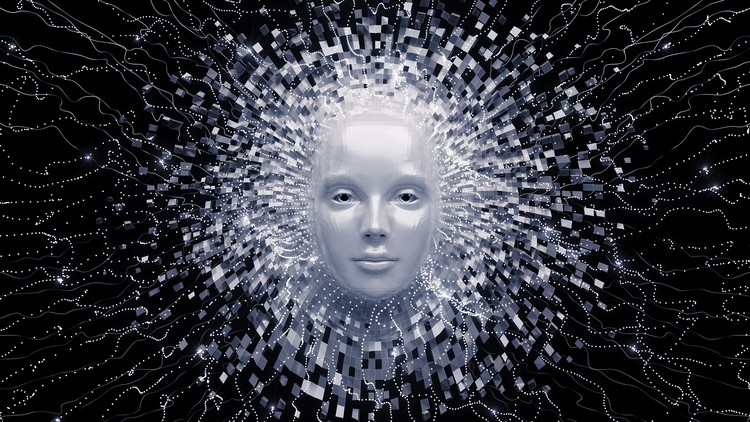